In JavaScript, null
is a special value that represents the intentional absence of any object value. It is a primitive data type, and it is often used to signify the absence of an object or the lack of a value where one is expected. null
is one of the primitive values in JavaScript, alongside undefined
, boolean
, number
, string
, and symbol
.
Checking for null
in JavaScript is important for several reasons, primarily related to avoiding errors and ensuring that your code behaves as expected. Here are some key reasons why checking for null
is crucial:
Preventing Null Reference Errors:
Attempting to access properties or methods on a variable that is null
or undefined
can result in a runtime error. Checking for null
before trying to access properties or methods helps prevent these null reference errors.
Ensuring Safe Operations:
When working with data that may or may not be present, checking for null
ensures that operations are performed only when the data is available. This is especially important when dealing with external data sources or user inputs.
Avoiding Unintended Falsy Conditions:
In conditional statements, it’s important to explicitly check for null
to avoid unintended falsy conditions. Falsy values include not only null
but also undefined
, false
, 0
, NaN
, and an empty string (''
).
Handling Optional Values:
In scenarios where a value is optional or may be missing, checking for null
helps you handle these cases gracefully. This is common when dealing with API responses or configuration options.
How to check for nulls
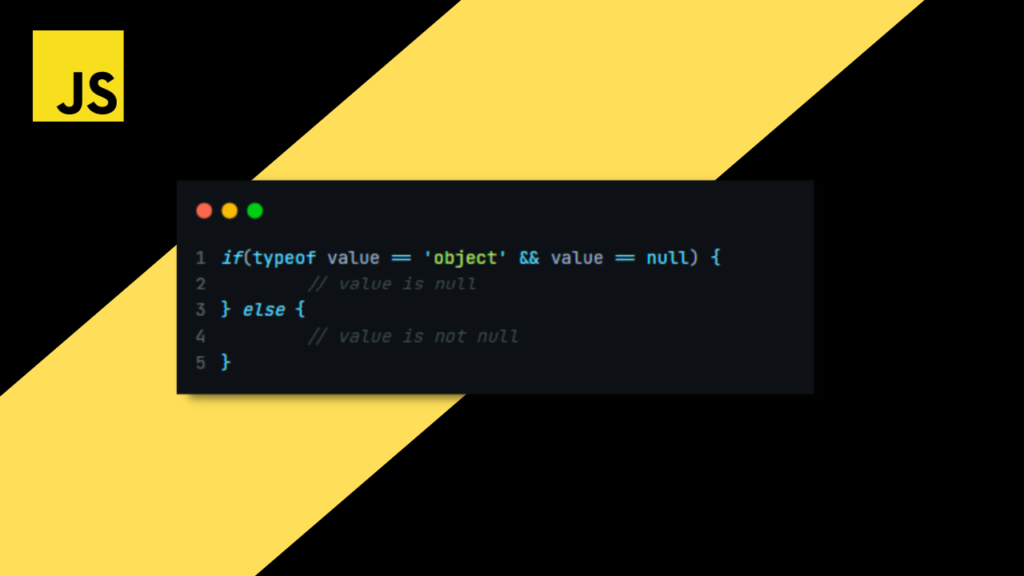
There are many ways to check for nulls in JavaScript. In this post, we will see four ways to check for nulls.
Methods to check for nulls
- Using strict equality operator
- Using nullish coalescing operator (??)
- Using typeof operator
- Using object.is()
Related Articles:
Method 1 – Using strict equality operator
The strict equality operator in JavaScript is denoted by ===
. It is used to compare two values for equality without performing type coercion. This means that not only must the values be equal, but they must also have the same data type.
if(value === null) {
// value is null
} else {
// value is not null
}
If block condition will pass only for null and will not pass if the value is “”, undefined, false, 0 or NaN.
Method 2 – Using nullish coalescing operator (??)
The nullish coalescing operator (??
) is a relatively recent addition to JavaScript, introduced with ECMAScript 2020 (ES11). It provides a concise way to handle the case where a value might be null
or undefined
, and you want to use a default value if the variable is nullish.
let value = null;
let result = value ?? "CodeyMaze";
If value is null, result will be set to CodeyMaze
Method 3 – Using typeof operator
The typeof
operator in JavaScript is a unary operator that is used to determine the data type of a given expression. It returns a string indicating the type of the operand.
if(typeof value == 'object' && value == null) {
// value is null
} else {
// value is not null
}
Using typeof method, first will check if the value is of type null, if yes then checking for null value.
Method 4 – Using object.is()
Object.is()
is a static method introduced in ECMAScript 2015 (ES6) to provide a more reliable way to compare values for equality in JavaScript. This method provides a strict equality comparison.
let value = null;
if(Object.is(value, null)) {
// value is null
} else {
// value is not null
}
Conclusion:
In this tutorial, we used four approaches to check for nulls in javascript.
In the first approach, we used a strict equality operator. In the second approach, we used nullish coalescing operator (??).
In the third approach, we used typeof with equality check. In the fourth approach, we make use of object.is() method.