GitHub Copilot is an AI coding assistant developed by GitHub and OpenAI. It can integrate with popular code editors like Visual Studio Code, Neovim, and JetBrains, which helps developers write code faster by suggesting whole lines or blocks of code based on context.
Install GitHub Copilot in VS code
If you don’t have Visual Studio installed, you can install it from the official website: VS code official website. To use GitHub copilot you need to have an account on GitHub. You may need a GitHub subscription, as Copilot is a paid service (though there’s a free trial for new users).
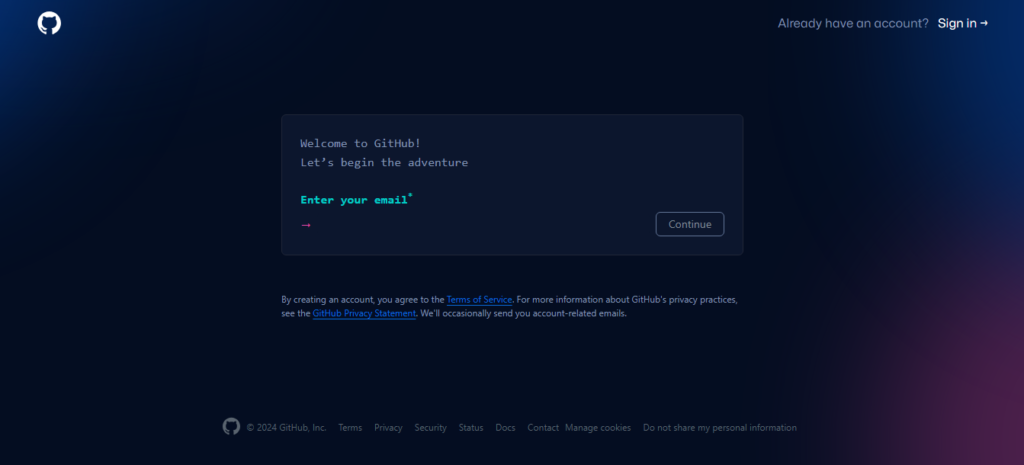
To install copilot in VS code, Follow these steps-
- Open Visual Studio Code
- Navigate to the Extensions panel on the left sidebar.
- In the search bar, type GitHub Copilot.
- Look for the official GitHub Copilot extension from GitHub and click Install.
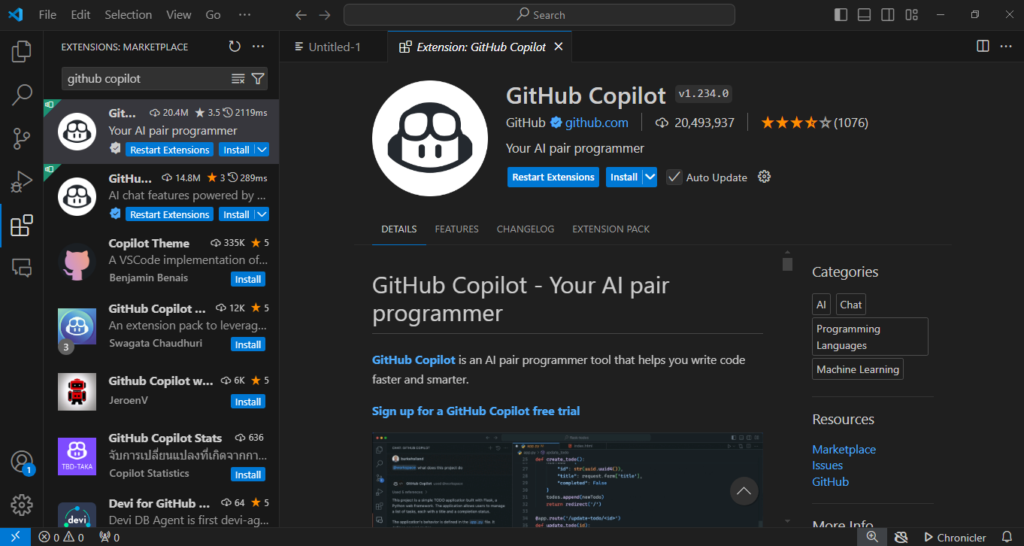
Once the extension is installed, you will be prompted to sign in to your GitHub account. After clicking Sign in, you’ll be redirected to GitHub’s website. Log in with your GitHub credentials. Approve the sign-in, and you will be redirected back to VS Code.
After signing in, GitHub Copilot should be active and ready for use in VS Code. That’s all you have successfully installed GitHub copilot.
How does GitHub Copilot provide code suggestions?
GitHub Copilot offers coding suggestions as you type. For example, you are working on a JavaScript file and want to write a function that checks if a number is prime. You start by typing :
function isPrime(number) {
As you type, GitHub Copilot suggests the entire function body:
function isPrime(number) {
if (number <= 1) return false;
for (let i = 2; i < number; i++) {
if (number % i === 0) return false;
}
return true;
}
You can press Tab to accept this suggestion. Press Esc if you want to dismiss it and Use Ctrl + Enter to open a dedicated Copilot pane with multiple suggestions.
Example 1: Generating a Python Docstring
You are working on a Python function and want to document it. GitHub Copilot can help generate a proper docstring automatically. Start typing the function:
def add_numbers(a, b):
return a + b
Now, you want to add a docstring to this function. Place the cursor below the function declaration and type """
. Copilot will suggest a complete docstring:
"""
Adds two numbers together.
Args:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of a and b.
"""
You can press Tab to accept the docstring, which documents the function’s purpose and parameters.
Example 2: Writing Unit Tests
Suppose you are using Copilot to write a test for your JavaScript function. You start typing the following:
test('isPrime should return false for non-prime numbers', () => {
GitHub Copilot suggests:
test('isPrime should return false for non-prime numbers', () => {
expect(isPrime(4)).toBe(false);
expect(isPrime(9)).toBe(false);
expect(isPrime(1)).toBe(false);
});
Copilot automatically generates relevant test cases based on the function name and intent, helping you write tests more efficiently.
The above examples show us how GitHub copilot can assist with code generation, documentation, and testing.