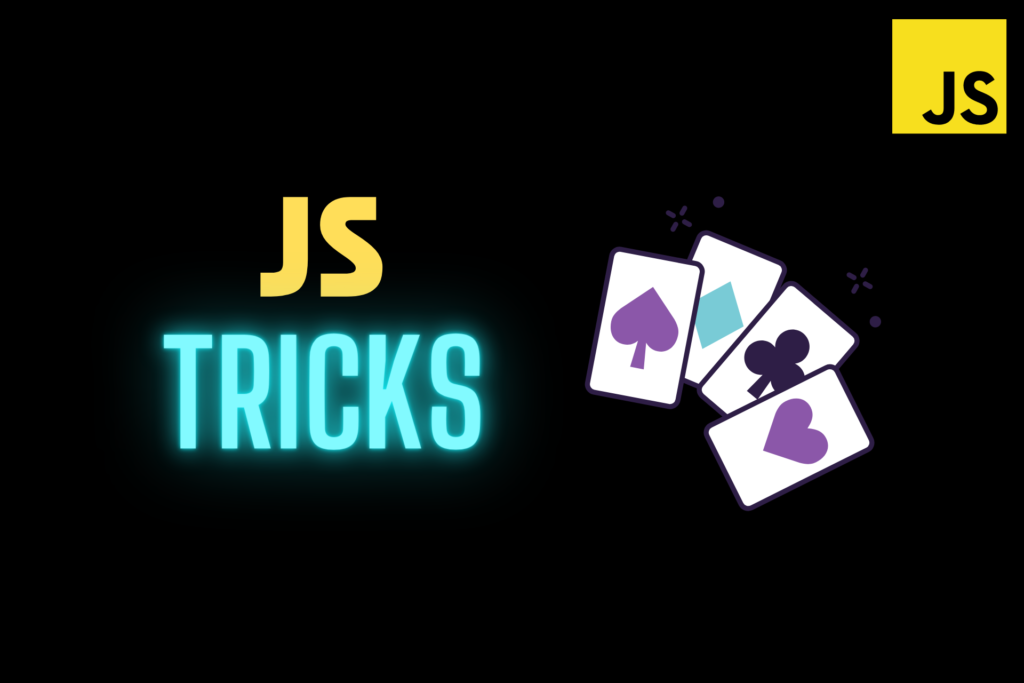
JavaScript is a popular programming language that is widely used for front-end web development, server-side scripting, and creating mobile and desktop applications.
In this article, we will discuss some useful JavaScript tricks that can help you write better code and improve your skills as a developer.
Convert Array to Object using Spread Operator
The spread operator can be used to copy the elements of an array into a new object.
const array = ['a', 'b', 'c', 'd', 'e'];
const object = { ...array };
console.log(object); // Outputs: { 0: 'a', 1: 'b', 2: 'c', 3: 'd', 4: 'e' }
Fill Array with Data
The Array.from
method creates a new array from an array-like or iterable object. You can use it to create an array with a specific length and fill it with a default value or based on a mapping function:
const length = 5; // Desired length of the array
const filledArray = Array.from({ length }, (_, index) => index);
console.log(filledArray); // Outputs: [0, 1, 2, 3, 4]
The fill
method fills all the elements of an array with a specified value. If you want to fill an array with a specific value, you can use this method:
const length = 5; // Desired length of the array
const fillValue = 'default';
const filledArray = new Array(length).fill(fillValue);
console.log(filledArray); // Outputs: ['default', 'default', 'default', 'default', 'default']
Merge Arrays using Spread Operator
You can use the spread operator to merge arrays in JavaScript. The spread operator allows you to expand the elements of an array into a new array. Here’s how you can use it to merge arrays:
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const mergedArray = [...array1, ...array2];
console.log(mergedArray); // [1, 2, 3, 4, 5, 6]
In this example, the spread operator …
is used to spread the elements of array1 and array2 into the mergedArray. This creates a new array containing all the elements from both arrays.
Convert Number to String use toString()
The toString()
method is used to convert a number into its corresponding string representation. This method is available for all primitive number types, including integers, floating-point numbers, and special numeric values like NaN and Infinity.
let integerNumber = 42;
let integerString = integerNumber.toString();
console.log(integerString); // Outputs: "42"
let nanValue = NaN;
let nanString = nanValue.toString();
console.log(nanString); // Outputs: "NaN"
Using && Operator
&& Operator is used to combine two or more expressions and returns true if all the expressions evaluate to true, otherwise, it returns false.
let isLoggedIn = true;
isLoggedIn && 'User is logged in!'