It is essential for any frontend developer to have a thorough understanding of CSS as it is fundamental in creating visually attractive and responsive web applications. When preparing for frontend interviews, it is important to have a comprehensive understanding of concepts like selectors, layout techniques, & responsive design principles.
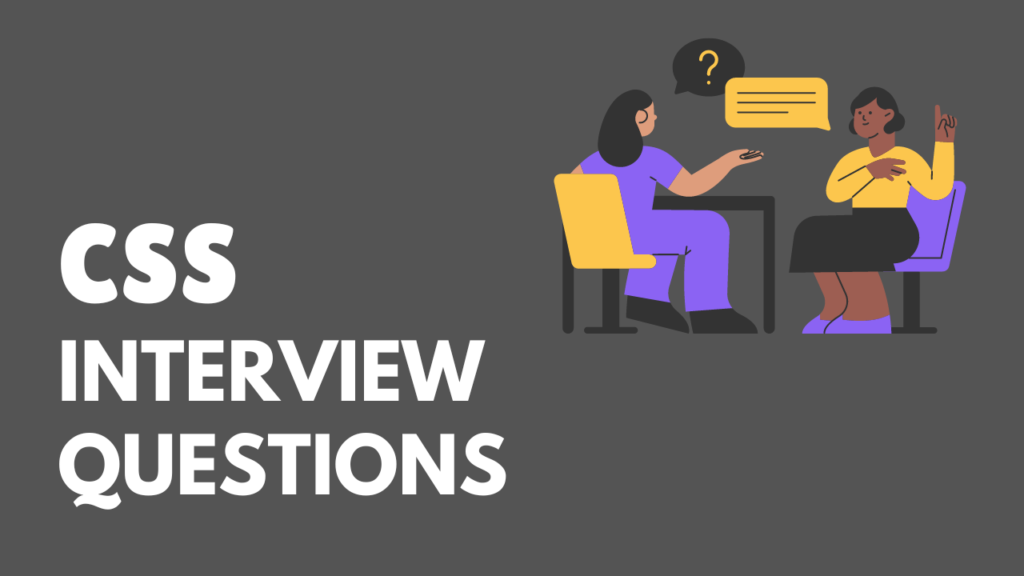
In this article, we have created a list of CSS interview questions that will be helpful for both beginners and experienced frontend interviews.
1. What is CSS? Why it is used?
CSS stands for Cascading Stylesheets, it is a language used to define the formatting and layout of HTML elements. To attach the CSS with the HTML document, we must include it in the HTML document.
2. How to include CSS on a web page?
There are 3 ways to include CSS on a web page-
- Inline, used to style only a small piece of code
<p style="color:red"> codeymaze </p>
- Embedded, style sheets are put between the style tags
<style> p{color:red} </style>
- External
<link rel="stylesheet" type="text/css" href="style.css">
3. What is the difference between padding and margin in CSS?
In CSS, padding and margin both are used to create space around an element. Padding defines the space inside an element, between content and border. Margin defines the space outside of the element, between the element and its surrounding elements.
4. What are pseudo-classes
in CSS?
Pseudo-classes are special keywords in CSS that allow you to apply styles to elements based not only on their state or position in the document tree but also on user interaction. Eg. :hover, :checked, :first-child.
5. What is the CSS Box model and what are its elements?
The Box Model is the foundational concept in CSS that describes the structure of an HTML element. It encompasses four key components: content, padding, border, and margin.
- Margin – The amount of space between the border and any surrounding element
- Border – A border surrounding the padding (if any) and content
- Padding – The amount of space between the border and the content
- Content – The content of the box, where text and images appear
6. What is the difference between width: auto
and width: 100%
?
width:auto
will try as hard as possible to keep an element the same width as it’s parent container when additional space is added from margins, padding, or borders.
width:100%
will make the element as wide as the parent container. Extra spacing will be added to the elements size without regards to the parent. This typically causes problems.
7. What does margin: auto
do?
You can set the left and right margins to auto
to horizontally center an element within its container.
8. What is CSS Grid? How do you create a CSS grid layout?
CSS Grid Layout allows for the creation of complex grid-based layouts with ease.
Basic steps include:
- Position grid items using properties like
grid-column
andgrid-row
. - Define a grid container with
display: grid
. - Define rows and columns with
grid-template-rows
andgrid-template-columns
.
.container {
display: grid;
grid-template-columns: 1fr 1fr 1fr;
grid-template-rows: auto;
}
.item {
grid-column: 1 / 3;
grid-row: 2;
}
9. What are CSS variables and How do you use them?
CSS variables allow you to store values that can be reused through out your stylesheet. They are defined using the --prefix
and accessed using the var()
function.
:root {
--main-color: #3498db;
--padding: 16px;
}
.element {
color: var(--main-color);
padding: var(--padding);
}
10. Explain the :root
pseudo-class and its usage
The :root
pseudo-class matches the document’s root element (usually <html>
). It is commonly used to define global CSS variables.
:root {
--main-bg-color: #f0f0f0;
}
body {
background-color: var(--main-bg-color);
}
11. Explain the use of the z-index
property in CSS.
z-index property specifies the stack order of elements. Elements with higher z-index are stacked above those with a lower z-index. It only works for positioned elements (elements with position set to absolute
, fixed
, relative
or sticky
)
12. What does * { box-sizing: border-box; } do?
By default elements have box-sizing: content-box
applied & only the content size if being accounted for. box-sizing: border-box
changes how the width and height are being calculated, border and padding are also being included in the calculation.
13. What’s the difference between a relative
, fixed
, absolute
& statically
positioned element?
- Relative : The element’s position is adjusted related to itself, without changing the layout.
- Static : This is the default position, the element will flow on the page as it normally would.
- Fixed : The element is positioned at a specified position relative to viewport and does not move when scrolled.
- Absolute : The element is positioned at a specified position relative to its closest positioned ancestor. Absolutely positioned elements do not affect the position of other elements.
14. How do you achieve responsive design using CSS?
We can achieve responsive design in CSS using various techniques, some of them includes :
- Media Queries : apply styles based on device width like
@media (max-width: 600px){ }
- Flexible Grid Layouts : use percentage for width like
width: 50%
15. Explain the concept of specificity in CSS. How is it calculated?
Specificity determines which CSS rule is applied by the browser when multiple rules match the same element. It is calculated based on:
- Inline styles:
1000
(highest specificity) - IDs:
0100
- Classes, attributes, and pseudo-classes:
0010
- Elements and pseudo-elements:
0001
- Universal selectors (
*
) and combinators:0000
(lowest specificity)
16. Explain CSS Flexbox.
Flexbox is a layout module designed for arranging elements in a one-dimensional space (row or column).
display:flex
: Defines a flex container.flex-direction
: Defines the direction of the flex items (row, column).justify-content
: Aligns flex items along the main axis (start, center, space-between, space-around).align-items
: Aligns flex items along the cross axis (start, center, stretch).flex-wrap
: Allows flex items to wrap onto multiple lines (nowrap, wrap, wrap-reverse).
17. How CSS Grid layout is different from Flexbox?
CSS Grid is for two-dimensional layout system [Rows & Columns] and flexbox is for one-dimensional layouts [Either a row or a column].
18. How can you center a div vertically and horizontally using CSS?
Using Flexbox :
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh; /* Full viewport height */
}
Using CSS Grid :
.container {
display: grid;
place-items: center;
height: 100vh;
}
19. How would you select all direct children elements of a particular type?
To select all direct children elements of a particular type, you can use >
child selector combined with the desired element to build the selector.
For ex to select all the direct children of <ul> element, you can use
ul > li {
/* Styles here */
}
20. How can you clear sides of a floating element?
We can add the clear
property to the element that needs to clear the float. The clear
property specifies on which sides of an element other floating elements are not allowed to float.
The possible values for the clear
property are:
none
: Allows floating elements on both sides (default).left
: No floating elements allowed on the left side.right
: No floating elements allowed on the right side.both
: No floating elements allowed on either side.
21. What is the difference between pseudo-classes and pseudo-elements in CSS?
A pseudo-class is a selector that selects existing elements that are in a specific state, e.g. hovered over, checked, focused, etc. Pseudo-classes start with a colon :
. Some common pseudo-classes are :active
, :checked
, :enabled
, :first-child
, :first-of-type
, :focus
, :hover
, :last-child
, :last-of-type
, :nth-of-type
, :visited
, etc.
post a:hover {
font-size: 120%;
font-weight: bold;
}
Pseudo-elements behave in a similar way, however they act as if you had added a whole new HTML element into the markup, rather than applying a class to existing elements. Pseudo-elements start with a double colon ::
. Most common pseudo-elements are ::after
, ::before
, ::first-letter
, and ::first-line
.
post p::first-line {
font-size: 120%;
font-weight: bold;
}
22. Explain universal selector (*
)
The universal selector (*
) in CSS is a wildcard selector that matches any element in the document. This is also known as the universal reset.
* {
padding: 0;
margin: 0;
}
In this example, the universal selector (*
) resets the margins and padding of all HTML elements to 0.
23. What is the overflow property in CSS used for?
The overflow property in CSS is used to control how content that overflow an element’s box is handled. This property specifies whether to clip content or to add scrollbars when an element’s content is too big to fit in a specified area. Below are the overflow options available in CSS –
visible
(default): The overflow is not clipped. The content renders outside the element’s box if it is too large.hidden
: The overflow is clipped, and the rest of the content will be invisible.scroll
: The overflow is clipped, but a scrollbar is added to see the rest of the content.auto
: Similar toscroll
, but the scrollbar is only added if the content overflows.
24. What is the difference between flex-grow and flex-shrink properties in Flexbox?
flex-grow
is a property in Flexbox that specifies how much a flex item should grow relative to the other items in the container, while flex-shrink
specifies how much a flex item should shrink relative to the other items when there is not enough space available in the container.
25. What is the difference between fluid and fixed layouts in CSS?
A fluid layout in CSS adjusts its width and height based on the size of the screen, while a fixed layout has a set width and height. Fluid layouts use percentages to set their dimensions, while fixed layouts use pixels.
26. What is the difference between min-width and max-width in CSS media queries?
In CSS media queries, min-width sets the minimum screen width at which a set of styles will be applied, while max-width sets the maximum screen width at which a set of styles will be applied.
For example, if you use min-width: 768px, the styles will only be applied to screens that are 768 pixels wide or larger, while if you use max-width: 768px, the styles will only be applied to screens that are 768 pixels wide or smaller.
27. What is the difference between responsive and adaptive design in CSS?
Responsive design in CSS adapts to different screen sizes and devices by using flexible grids, fluid images, and media queries to adjust the layout and content of the website. This approach focuses on creating a single layout that scales and rearranges itself to fit different screen sizes.
Adaptive design in CSS, on the other hand, uses predefined layout sizes and breakpoints to adjust the layout and content based on the screen size and device being used. The server or client-side script detects the user’s device and delivers the appropriate layout.
28. What is the purpose of CSS content property?
The CSS content property is used to insert content before or after an HTML element using the ::before and ::after pseudo-elements.
29. What is SASS?
Sass is a CSS preprocessor that adds functionality to CSS, such as variables, nesting, and more. It allows us to write more efficient code and simplifies task like browser compatibility.
30. what is the difference between sass and SCSS?
Sass and SCSS are both CSS pre-processors and are very similar, but they have different syntax. SCSS is the main syntax for SASS, which is a build on top of the CSS syntax that already exists.
Sass has a more concise and less verbose syntax, with no curly braces and no semicolon whereas SCSS has a syntax that is almost identical to standard CSS, with curly braces and semicolons.