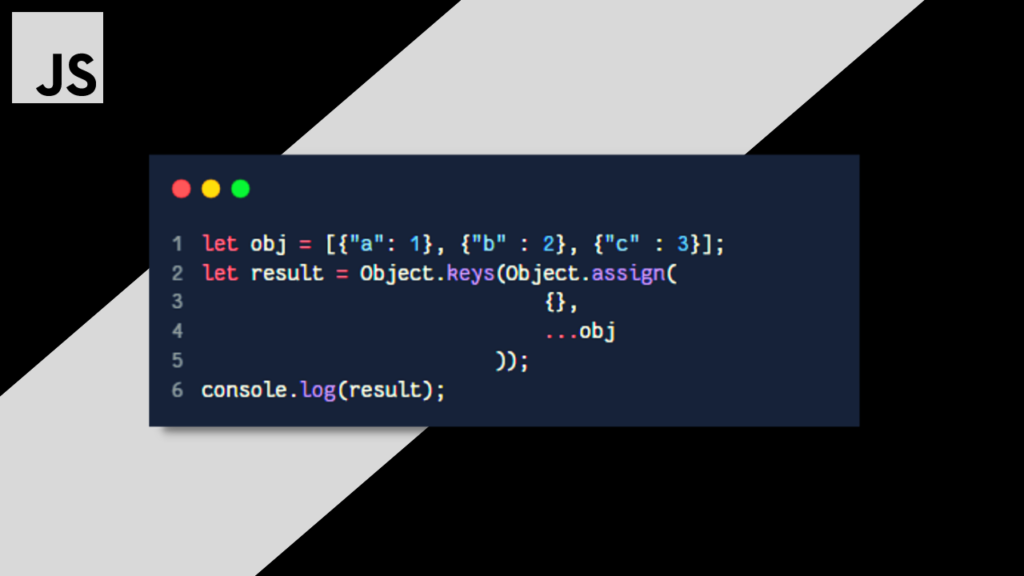
You can get the keys of each object inside an array of objects in many ways. In this article, we will cover two methods.
Methods to get the keys of each object
- Using Object.keys() and Object.assign()
- Using Array.flatMap() and Object.keys()
Related Articles:
Method 1: Using Object.keys() and Object.assign()
Object.keys() – Object.keys() is a javascript method that returns an array of the enumerable property names of the given object. In other words, it extracts the keys of an object and returns them as an array. The order of the keys in the array is determined by the order in which they are defined in the object.
Object.assign() – Object.assign() is a method in JavaScript used to copy the values of all enumerable properties from one or more source objects to a target object. It returns the modified target object.
let obj = [{"a": 1}, {"b" : 2}, {"c" : 3}];
let result = Object.keys(Object.assign(
{},
...obj
));
console.log(result);
The Output of the above code will be
(3) ["a", "b", "c"]
0:"a"
1:"b"
2:"c"
[[Prototype]]:[]
Method 2: Using Array.flatMap() and Object.keys()
Array.flatMap() – Array.flatMap() is a method in JavaScript that both maps and flattens an array. It applies a provided mapping function to each array element and then flattens the result into a new array.
let obj = [{"a": 1}, {"b" : 2}, {"c" : 3}];
let result = obj.flatMap(Object.keys);
console.log(result);
The Output of the above code will be
(3) ["a", "b", "c"]
0:"a"
1:"b"
2:"c"
[[Prototype]]:[]
You can always choose whichever is best for you, Thanks for reading!